A dramatic trial is currently underway at the Central Criminal Court, where two men, Sean kavanagh, 26, and Calvin Dunne, 24, are facing charges for the murder of 29-year-old Dylan McCarthy. The tragic incident, which occurred in August 2022 outside The Bellyard pub in Monasterevin, Co Kildare, has sparked intense scrutiny over the circumstances leading to McCarthy’s death.
Prosecuting counsel Seoirse Ó Dúnlaing presented the case, stating that mccarthy suffered a fatal brain injury after being punched and kicked during a violent altercation. Emergency services found McCarthy unresponsive at the scene, and he passed away the following day.A post-mortem examination revealed that a severed artery caused his traumatic brain injury.
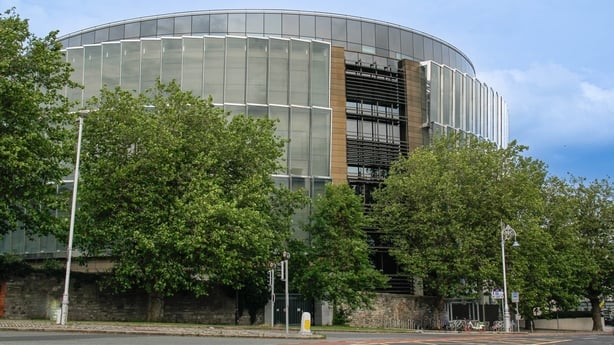
According to Mr. Ó Dúnlaing, the confrontation did not begin with the accused. McCarthy and his family were reportedly ejected from the pub following an internal dispute. A fight then broke out in the foyer, with Kavanagh and dunne allegedly engaging in an altercation with McCarthy outside the premises.
the prosecution alleges that Kavanagh delivered multiple blows to McCarthy, after which Dunne punched him with such force that he fell to the ground. Dunne then allegedly kicked McCarthy in the head,an act described as “vicious” by the counsel. These actions, the prosecution argues, went far beyond self-defense.
Mr. Ó Dúnlaing emphasized the distinction between self-defense and excessive force, stating, “There was a difference between defending yourself and having a straightener.” He urged the jury to consider whether the defendants intended to cause serious harm or death, noting that such intentions can be formed in an instant.
In addition to the murder charge, kavanagh is accused of assaulting Dylan’s father, Eamon McCarthy, causing him harm. Both men are also charged with violent disorder. The trial, expected to last two weeks, will explore the complex events surrounding the fatal incident.
As the jury evaluates the evidence, the case raises critical questions about the limits of self-defense and the devastating impact of unchecked violence.
What are some best practices for using null in programming?
Table of Contents
- 1. What are some best practices for using null in programming?
- 2. Understanding Null in Programming: Best Practices and Implementation
- 3. What is Null in Programming?
- 4. How is Null Implemented Across programming languages?
- 5. Best Practices for Using Null in Code
- 6. Example Use Case: Handling Null in a Real-World Scenario
- 7. Conclusion
- 8. Mastering NULL Checks in C: A Guide to Writing Robust Code
- 9. Why NULL Checks Matter
- 10. When to Check for NULL
- 11. Example Use Case
- 12. Best Practices for Handling NULL
- 13. Conclusion
- 14. What are the potential consequences of not performing NULL checks when working with pointers in C?
- 15. Best Practices for NULL Checks
- 16. Practical Example: Handling NULL Pointers
- 17. conclusion
Table of Contents
Understanding Null in Programming: Best Practices and Implementation
What is Null in Programming?
In the world of programming, null is a unique value that signifies the absence of a valid object or meaningful data. It’s a cornerstone concept used to indicate that a variable or object doesn’t reference any memory location or hold any useful details. Think of it as a placeholder for “nothingness” in your code.
How is Null Implemented Across programming languages?
The way null is implemented can vary significantly depending on the programming language and its runtime environment. Here’s a breakdown:
- Memory Portrayal: In many systems, null is represented as an unused memory address, often
0x00000000
. This ensures it doesn’t point to any valid object or data. - Language-Specific Behaviour: Different languages handle null in unique ways. For instance:
- In Java or C#, null is used to denote an uninitialized or non-existent object.
- In C or C++, null is represented as a null pointer (
NULL
ornullptr
), which doesn’t point to any valid memory location. - In JavaScript, null is a primitive value explicitly representing the absence of an object value.
Best Practices for Using Null in Code
Effective use of null is essential to avoid common pitfalls like null pointer exceptions. Here are some tips to help you write cleaner, safer code:
- Initialization: Use null to initialize variables when you don’t yet have a valid value to assign.
- Null Checks: Always verify if a variable or object is null before using it to prevent runtime errors.
- Returning Null: Return null from functions or methods to indicate the absence of a valid result, but ensure this behavior is well-documented.
- Avoid Overuse: Overusing null can make your code harder to maintain. Consider alternatives like optional types (e.g.,
Optional
in Java orMaybe
in Haskell) to enhance code robustness and clarity.
Example Use Case: Handling Null in a Real-World Scenario
Imagine you’re building a user profile system. A user’s middle name is optional, so it might not always have a value. Here’s how you could handle this with null:
function getMiddleName(user) {
return user.middleName || null;
}
let middleName = getMiddleName(currentUser);
if (middleName === null) {
console.log("Middle name not provided.");
} else {
console.log("Middle name: " + middleName);
}
This approach ensures your code gracefully handles missing data without throwing errors.
Conclusion
Null is a powerful yet nuanced concept in programming. While it’s indispensable for representing the absence of data, its misuse can lead to bugs and maintenance challenges. By understanding its implementation and adhering to best practices, you can write more robust and maintainable code.Whether you’re initializing variables, checking for null, or exploring alternatives like optional types, mastering the use of null is a critical skill for every developer.
Mastering NULL Checks in C: A Guide to Writing Robust Code
When working with pointers in C, one of the most critical practices is ensuring they are properly checked for NULL values. This simple yet powerful technique can prevent your program from crashing or behaving unpredictably. But when exactly shoudl you perform these checks? Let’s dive into the details and explore how to handle NULL pointers effectively.
Why NULL Checks Matter
NULL pointers are a cornerstone of C programming, representing the absence of a valid memory address. While they can be useful in certain scenarios, failing to handle them correctly can lead to serious issues like segmentation faults or undefined behavior. As an example, dereferencing a NULL pointer is a common mistake that can crash your program instantly.
As one expert aptly put it, “When should pointers be checked for NULL in C? In that situation, you need to decide whether a NULL argument is legal (such as code that decides a null char* is treated the same as an empty string), and if it’s not, change the documentation, assert in the code, and fix all the problems; have the code reviewed and check it in.”
When to Check for NULL
Determining when to check for NULL depends on the context of your code. Here are some key situations where NULL checks are essential:
- Function Arguments: Before processing a pointer passed as an argument,verify it isn’t NULL. this ensures your function handles invalid inputs gracefully.
- dynamic Memory Allocation: Always check the return value of functions like
malloc()
orcalloc()
. If memory allocation fails, these functions return NULL. - Pointer Dereferencing: Never dereference a pointer without first confirming it points to a valid memory location.
- Function Returns: If a function returns a pointer,check its value before using it to avoid unintended behavior.
Example Use Case
Let’s look at a practical example. Suppose you’re writing a function to search for a user by their ID. Here’s how you might implement it:
java
public User findUserById(int id) {
// Logic to search for user
if (userExists) {
return user;
} else {
return null; // indicates user not found
}
}
When calling this function, always perform a NULL check before proceeding:
java
User user = findUserById(123);
if (user != null) {
// Use user object safely
} else {
System.out.println("User not found.");
}
This ensures your program handles the absence of a user gracefully,rather than crashing or producing errors.
Best Practices for Handling NULL
To write clean, reliable code, follow these best practices:
- Document Your Intentions: Clearly state whether a NULL pointer is a valid input for your functions.
- Use Assertions: In development, use assertions to catch NULL pointers early and debug issues.
- Leverage Static Analysis tools: Tools like lint or modern IDEs can definitely help identify potential NULL pointer issues during development.
- Code Reviews: Have your code reviewed by peers to ensure NULL checks are implemented consistently and correctly.
Conclusion
Understanding and using NULL correctly is essential for writing robust and error-free code. While it’s a powerful tool for representing the absence of a value, it must be handled with care to avoid common pitfalls. By incorporating NULL checks into your programming practices, you can create more reliable and maintainable applications.
For a deeper dive into the topic, you can refer to the full guide on freeCodeCamp.
What are the potential consequences of not performing NULL checks when working with pointers in C?
Racefully.
Hear are some tips to ensure your NULL checks are effective and maintainable: Let’s look at a simple example where NULL checks are critical. Suppose you’re writing a function to print a string: In this function, the NULL check ensures the program doesn’t crash if a NULL pointer is passed. Instead,it prints an error message and exits gracefully. NULL checks are a vital part of writing reliable and robust C code. By understanding when and how to perform these checks, you can prevent common pitfalls and ensure your program behaves predictably. Whether you’re working with function arguments, dynamic memory, or returned pointers, incorporating NULL checks into your coding practices will save you from headaches down the road. Remember, it’s better to be safe than sorry when dealing with pointers in C.malloc
,calloc
,or realloc
,always check if the returned pointer is NULL. If it is indeed, it indicates memory allocation failed, allowing you to handle the error appropriately.Best Practices for NULL Checks
assert
to catch NULL pointers early. This can help identify issues during growth.Practical Example: Handling NULL Pointers
void printString(const char* str) {
if (str == NULL) {
printf("Error: NULL pointer provided.n");
return;
}
printf("%sn", str);
}
conclusion